Using Autorelease Pool for Efficient Memory Management
Memory management is a critical aspect of software development, and in Swift, Automatic Reference Counting (ARC) takes care of most memory management tasks. However, in certain situations, particularly when dealing with a large number of temporary objects, it’s beneficial to have more control over memory allocation and deallocation. This is where autoreleasepool
in Swift comes into play.
What is Autorelease Pool?
An autorelease pool is a mechanism in Swift (and Objective-C) that allows developers to explicitly control the lifetime of objects. The primary use case for autorelease pools is to manage the memory of short-lived objects efficiently.
When you create an object in Swift, it is automatically retained, and its reference count is incremented. When the object is no longer needed, the reference count is decremented. The memory associated with the object is deallocated when the reference count becomes zero.
However, there are situations where objects are needed only temporarily, and waiting for the next automatic reference counting cycle might not be efficient. This is where autorelease pools come in. An autorelease pool is a region of code where you can explicitly state that you don’t need the objects created within that scope beyond it.
autoreleasepool {
// Code that creates and uses temporary objects
// These objects will be released when the pool is drained
}
The autoreleasepool
block defines a scope within which temporary objects can be managed more actively.
Use Cases
1. Loops and Iterations
Consider a scenario where you are performing a repetitive task within a loop, and each iteration creates temporary objects. Using an autorelease pool can help in releasing memory more frequently, rather than waiting for the loop to complete.
func processItems() {
for item in items {
autoreleasepool {
// Code that creates and uses temporary objects for each iteration
}
}
}
2. Resource-Intensive Operations
In resource-intensive operations, such as image processing or parsing large datasets, creating an autorelease pool can help manage memory more efficiently during the operation.
func processLargeDataSet() {
autoreleasepool {
// Code that processes a large dataset with temporary objects
}
}
3. Multithreading
When working with multithreading, autorelease pools can be particularly useful to manage memory in concurrent operations.
DispatchQueue.concurrentPerform(iterations: 10) { index in
autoreleasepool {
// Code that runs concurrently and creates temporary objects
}
}
Example: Image Processing
Let’s take a practical example of using autoreleasepool
in the context of image processing. Consider a function that applies a filter to each pixel of an image.
func applyFilterToImage(image: UIImage) -> UIImage {
autoreleasepool {
// Code that processes each pixel and creates temporary objects
}
// Return the processed image
}
In this scenario, an autorelease pool ensures that memory is released more frequently during the image processing operation, preventing the accumulation of unnecessary temporary objects.
Debugging and Optimization
While autoreleasepool
is a powerful tool, it's important to use it judiciously. Overusing autorelease pools can lead to increased overhead, negating the performance benefits. Therefore, it's recommended to profile your code using tools like Instruments in Xcode to identify memory issues.
When optimizing performance-critical code, consider using autorelease pools strategically, especially in loops or blocks where a large number of temporary objects are created. Measure the impact on memory usage and execution time to ensure that your optimizations are effective.
Conclusion
While Automatic Reference Counting (ARC) handles memory management for most situations in Swift, autorelease pools provide a mechanism for developers to have more control in specific scenarios. Understanding when and how to use autorelease pools is crucial for optimizing memory usage, especially in situations involving a large number of short-lived objects.
By incorporating autorelease pools into your Swift code, you can ensure that memory is efficiently managed, leading to better performance and a more responsive application. As always, it’s essential to strike a balance and use autorelease pools judiciously, focusing on scenarios where their benefits are most pronounced.
Thank you for reading until the end. If you have any questions or feedback, feel free to leave a comment below.
If you enjoyed reading this article, please press the clap button 👏 . Your support encourages me to share more of my experiences and write more articles like this. Follow me here on medium for more updates!
Happy coding! 😊👨💻👩💻
More from Ruslan Dzhafarov
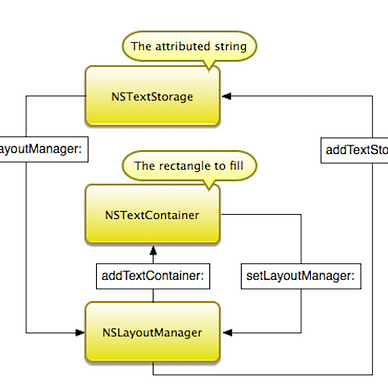
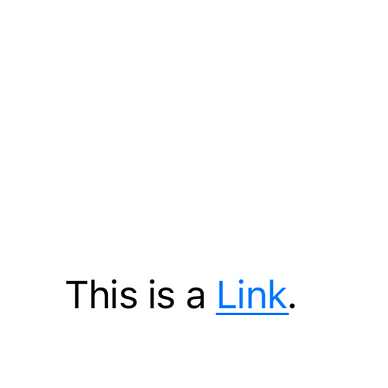
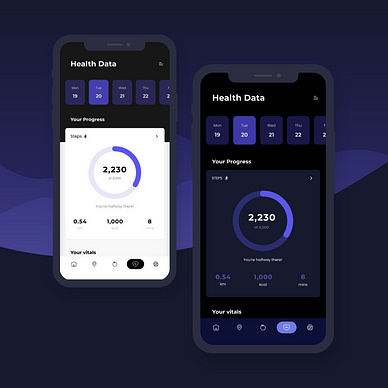
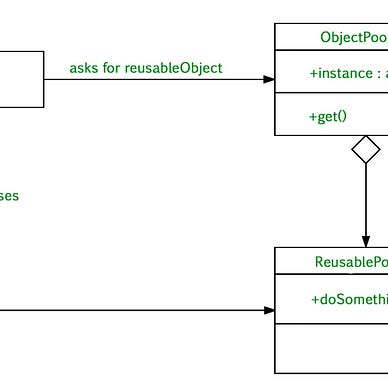
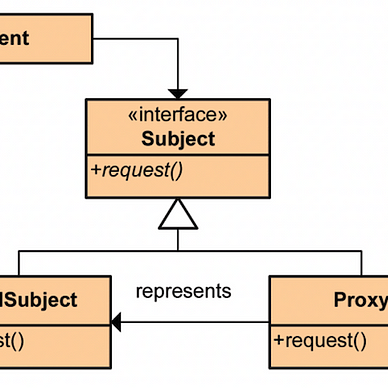
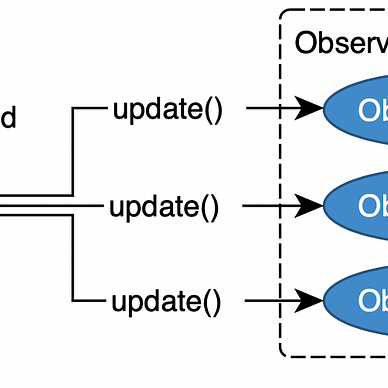
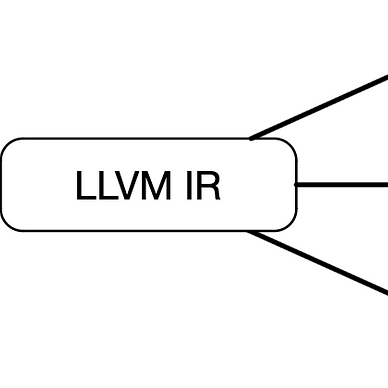
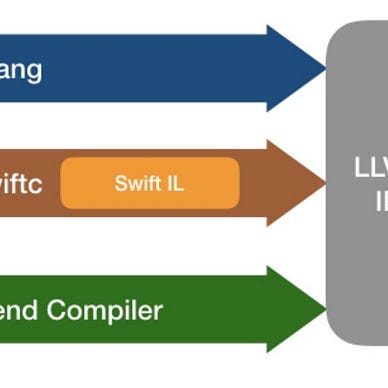
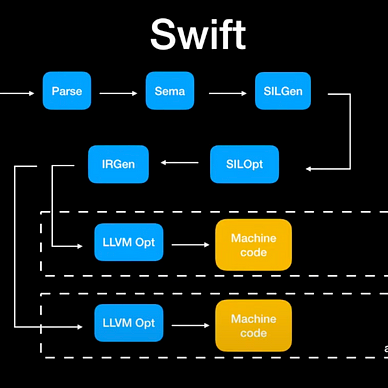