How to Upload images to Server Using multipart/form-data in iOS
When it comes to transmitting binary data, such as images, over the internet, using the right format is crucial for successful communication between clients and servers. One widely used format for this purpose is multipart/form-data
. This format allows us to send not only textual data but also binary data like images, making it an ideal choice for scenarios where file uploads are required. In this tutorial, we'll delve into the details of the multipart/form-data
format and explore how to leverage it for uploading images from an iOS application to a server.
What is multipart/form-data?
multipart/form-data
is a content type used in HTTP requests for sending binary data, files, and form data as a series of parts. Each part in the request body is separated by a boundary, and each part typically contains a header and a body. The header provides metadata about the part, such as the content type, while the body contains the actual data.
The key characteristics of multipart/form-data
include:
- Boundary: The boundary is a unique string that separates different parts within the request body. It ensures that each part is distinct and can be correctly identified by the server.
- Headers: Each part has headers that describe the content, including the content type and any other relevant information. These headers help the server interpret and process the incoming data correctly.
- Body: The body of each part contains the actual data being transmitted. This can include file content, form data, or any other binary information.
Why use multipart/form-data for Image Uploads?
When it comes to uploading images or files, multipart/form-data
is preferred for several reasons:
- Binary Data Support:
multipart/form-data
is designed to handle binary data efficiently, making it suitable for transmitting images and other file types. - Flexible Content: It supports sending a mix of text and binary data in a single HTTP request, allowing for versatile communication between clients and servers.
- Compatibility: Many server-side technologies and frameworks are equipped to handle
multipart/form-data
requests, making it a widely accepted and interoperable format.
In the next section of this tutorial, we will explore how to implement image uploads using the multipart/form-data
format in Swift, providing step-by-step guidance for iOS developers.
Implementing Image Upload Using multipart/form-data in Swift
Now that we have a fundamental understanding of the multipart/form-data
format, let's dive into the practical implementation of uploading images from an iOS Swift application to a server.
Step 1: Convert Image to Data
Before sending the image to the server, convert it to Data
using the jpegData
or pngData
method:
guard let imageData = image.jpegData(compressionQuality: 0.8) else {
// Handle error if unable to convert image to data
return
}
Step 2: Create the URLRequest
Construct a URLRequest
with the server endpoint URL and set the HTTP method to POST
:
guard let url = URL(string: "YOUR_SERVER_ENDPOINT_URL") else {
// Handle error if the URL is invalid
return
}
var request = URLRequest(url: url)
request.httpMethod = "POST"
Step 3: Set Up the Request Body
Build the request body using the multipart/form-data
format. Add the image data to the body using a unique boundary:
let boundary = UUID().uuidString
let clrf = "\r\n"
request.setValue("multipart/form-data; boundary=\(boundary)", forHTTPHeaderField: "Content-Type")
var body = Data()
body.append("--\(boundary)")
body.append(clrf)
body.append("Content-Disposition: form-data; name=\"file\"; filename=\"image.jpg\"")
body.append(clrf)
body.append("Content-Type: image/jpeg")
body.append(clrf)
body.append(clrf)
body.append(imageData)
body.append(clrf)
body.append("--\(boundary)--")
body.append(clrf)
request.httpBody = body
extension Data {
mutating func append(_ string: String) {
if let data = string.data(using: .utf8) {
append(data)
}
}
}
Step 4: Create URLSession Task for Upload
Generate a URLSession
task to send the request to the server:
let task = URLSession.shared.dataTask(with: request) { (data, response, error) in
// Handle the server response here
if let error = error {
print("Error: \(error.localizedDescription)")
return
}
// Process the response data
if let data = data {
let responseString = String(data: data, encoding: .utf8)
print("Response: \(responseString ?? "")")
}
}
// Start the URLSession task
task.resume()
Conclusion
In summary, this tutorial equipped you with the essentials for uploading images from iOS apps to servers using the multipart/form-data
format. With a focus on format fundamentals, implementation steps, and advanced considerations, you're now ready to integrate robust image upload functionality into your projects, ensuring a smooth user experience.
Thank you for reading until the end. If you have any questions, please feel free to write them in the comments.
If you enjoyed reading this article, please press the clap button 👏 . Your support encourages me to share more of my experiences and write more articles like this. Follow me here on medium for more updates!
Happy coding! 😊👨💻👩💻
More from Ruslan Dzhafarov
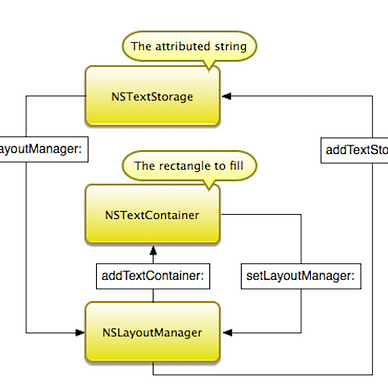
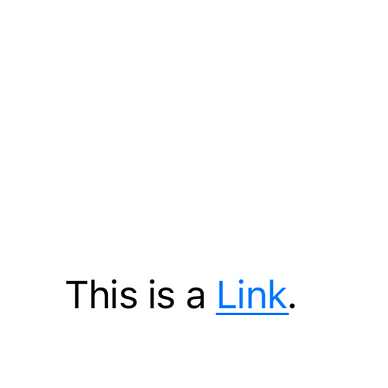
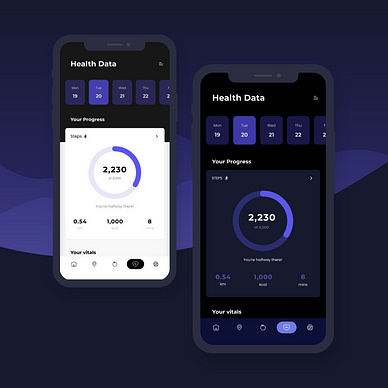
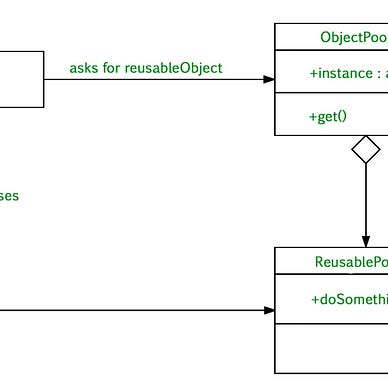
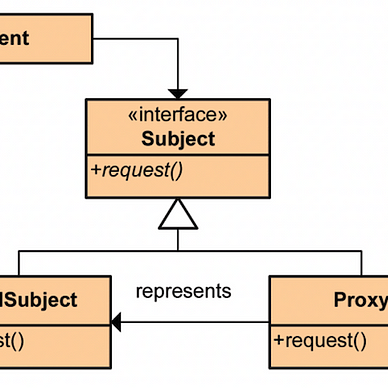
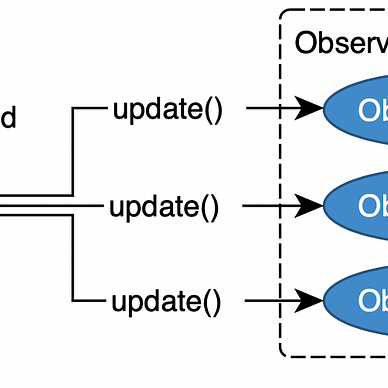
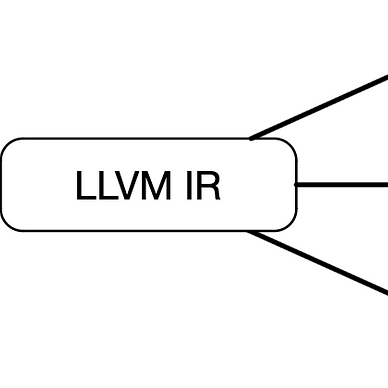
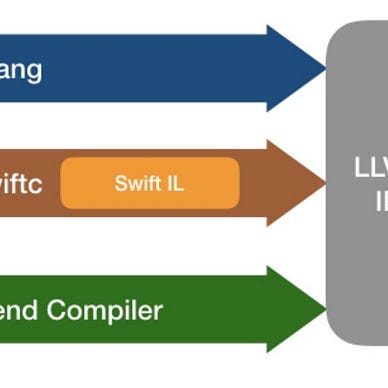
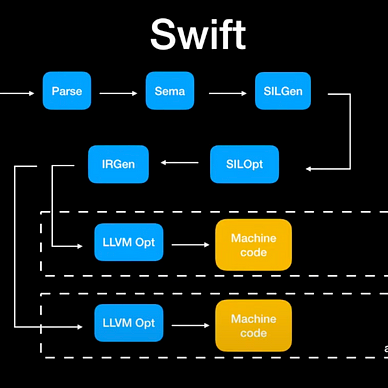